Understanding Kotlin Constructors and Initializers
What I really love about Kotlin is the elegance of its syntax! Everything is dynamic and concise! You can just look at how a Kotlin constructor is defined and you will be amazed! It seems a little bit magical to me compared to Java!
In this article, we will try to highlight and explain in depth the concept of constructors in Kotlin! We will cover everything you need to know about constructors to help you boost your learning process.
So, let’s get started.
What are the types of Kotlin constructors?
A constructor is at the heart of OOP, it describes a special member method – which has the name of the class – used mainly to initialize a class properties.
As the name suggests, a constructor is used primarily to construct or create an instance of your class.
Every class needs to have a constructor! If you don’t declare or define one, then the compiler generates a default constructor for you.
In Kotlin, a class can have one primary constructor and zero or more additional secondary constructors.
Primary constructor:
In short, primary constructor provide a simple way to initialize the member properties of a class. It takes a list of comma-separated parameters and declared just after the class name as a part of the header.
In Java, a parameterized constructor does not provide any actual software value to our program: You are basically saying that the parameters are only used to initialize properties of the same name and type in the body of the constructor. That’s all!
This is an example of how you declare a constructor in Java:
public class User {
private String login;
private String password;
public User(String login, String password) {
this.login = login;
this.password = password;
}
}
Well, in Kotlin, things are very different! Let’s take a look at this basic example of the same User class we already defined in Java:
class User constructor(login: String, password: String)
We can omit the constructor keyword if the primary kotlin constructor is not qualified by any annotations or visibility modifiers!
class User (login: String, password: String)
Yes, I know! Too much readability and visibility and this is what I like the most about Kotlin!
Well, Let me explain in detail what we did in the above example:
We used the keyword class to declare a class in Kotlin! So nothing new until now!
All the class properties are directly filled in parentheses, just after the name of the class! So maybe that’s something new!
The parentheses actually define what is known as primary constructor of the class User!
And finally, we didn’t have to declare the properties and the constructor body in our class. Awesome!
Very good! But are you sure the example in Kotlin represents exactly the one in Java? I don’t see the keyword public?
Good question! Yes, we created exactly the same Java class in Kotlin! In fact, the default visibility of any element of your Kotlin code (variables, functions, classes) is public!
Now let’s see together how we can create an object in Kotlin :
val user = User("admin","pass@2020")
Yes, no need for new operator to instantiate a class in Kotlin, or even the ; to end an instruction!
Default values in Kotlin constructor
Sometimes, we want our code to be more flexible and reusable to handle default values for optional parameters in our constructors!
Java by design does not support optional parameters! Fortunately, Kotlin unlike Java, provide built-in support for this concept! Kotlin provide a clean and concise syntax to define default parameters for constructors!
The concept is as obvious as it sounds. You can provide default values to parameters of the constructor!
You basically don’t have to explicitly define values for optional parameters during objects creation. The default values are used if you don’t pass any values for optional parameters while instantiating you class.
To make it simpler to understand, consider the following example:
class Employee (val firstname:String = "John", val lastname: String = "Smith"){
fun print() {
println("Hello "+this.firstname+" "+this.lastname)
}
}
fun main() {
val emp01 = Employee()
emp01.print()
val emp02 = Employee(firstname="Linda")
emp02.print()
}
**... and the output is:**
Hello John Smith
Hello Linda Smith
Let me explain a little bit what exactly did in the above example!
When creating the first Employee object emp01, we didn’t pass any values to the constructor, so the object is created with the default parameters values specified in primary constructor : “John” and “Smith”.
For the second object emp02, we have passed only the firstname value, so the lastname is initialized with its default value.
As you can see, default parameters in kotlin constructor can really make your code more readable, clean and flexible!
Primary constructor on the JVM
If all your primary constructor parameters have default values, then the compiler will automatically generate an another default constructor without parameters which will use the default values to create objects.
According to Kotlinlang documentation, this makes it easier to use Kotlin with libraries such as Jackson or JPA that create class instances through no args constructors.
@JvmOverloads annotation provide another way to generate multiple constructors based on number of the default arguments defined in the constructor.
If the constructor has 2 parameters with default values, then two constructors are generated for you! Awesome, right ?
The first constructor takes all the parameters except the first parameter which has a default value, the second takes all parameters except the two ones with default values!
Let’s try to update Employee class of the previous example with @JvmOverloads annotation:
class Employee @JvmOverloads constructor (val firstname:String = "John", val lastname: String = "Smith"){
fun display() {
println("Displaying "+this.firstname+" "+this.lastname)
}
}
fun main() {
val emp01 = Employee()
emp01.display()
val emp02 = Employee("WILLIAM")
emp02.display()
val emp03 = Employee("Alice","Johnson")
emp03.display()
}
**... and the output is:**
Displaying John Smith
Displaying WILLIAM Smith
Displaying Alice Johnson
We cannot omit the constructor keyword because it is annotated with @JvmOverloads annotation!
Kotlin init block
Primary Kotlin constructor has a constrained syntax, it is defined only to declare class properties, it does not accept any additional logic or code!
So, to fill this deficit, Kotlin provide another concise and flexible concept to allow us write some custom code: init block!
The init block define a place where you can add more custom code to perform some logic! The particularity of init block is that it is executed immediately after the primary constructor.
For me, initializer blocks are just some sort of extensions of the primary constructor!
Initializer block with example
We will try to understand init block concept with an example!
Let’s create a Person class with one primary constructor and two initializer block:
class Person (var name:String, var age: Int){
init {
println("Your first init block")
}
init {
name = "Alice"
age = 23
println("Init block : ${name} ${age}")
}
fun display(){
print("Display Function : ${name} ${age}")
}
}
fun main() {
val pers = Person("Linda",40)
pers.display()
}
//
Your first init block
Init block : Nicole 23
Display Function : Nicole 23
As you can see, we created a Person instance with name “Linda” and age 40, however both class properties are initialized with the values specified in the second initializer!
The following are some key points to keep in mind about init block:
A Kotlin class can contain one or more initializer blocks! They will be executed sequentially in the same order.
Init block is executed every time an instance is created.
initializer block is executed after the primary constructor is called and before any secondary constructors.
Secondary constructor:
Kotlin started to support the concept of secondary constructor since the release of M11 (0.11.)! Just after M11, a class can also declare one or more constructors called secondary constructors! They must be prefixed by the keyword constructor.
A Kotlin class can have zero or more secondary constructors! Every secondary constructor must call explicitly the primary constructor! You can do that by using this keyword! If you fail to do it, the compiler will simply complain: Primary constructor call expected!
You can also call another secondary constructor of the same class which calls directly the primary constructor!
You can’t declare class properties inside secondary constructor the same way we do in primary constructor! If you want to use some class variables inside the secondary constructor then you have to declare them inside the class!
Create multiple constructors in Kotlin
Sometimes, we need to define several constructors in the same class to customize the logic of how an object is created! So to achieve this, Kotlin provide the concept of multiple secondary constructors.
Let’s create a simple kotlin class with some secondary constructors!
class Student (var name:String, var age: Int){
// First secondary constructor
constructor (name:String) : this(name,20){
println("${name} ${age}")
}
// Second secondary constructor
constructor (age: Int) : this("Linda",age){
println("${name} ${age}")
}
fun display(){
println("Hello : ${name} ${age}")
}
}
fun main() {
val std01 = Student("Jonathan")
std01.display()
val std02 = Student(23)
std02.display()
}
// Program output:
Jonathan 20
Hello : Jonathan 20
Linda 23
Hello : Linda 23
In practice, secondary kotlin constructors will be mainly used in certain specific situations, in particular in case of inheritance to guarantee the interoperability between a parent class written in Java having multiple constructors, and a child class written in Kotlin.
Let’s take an example of a class that extends the parent class View:
class MycustomView : View {
constructor(context:Context?) : super(context)
constructor(context:Context?,attrs: AttributeSet?) : super(context,attrs)
}
As you can see, in this example we defined three secondary constructors in MycustomView class just to “match” with the different constructors of View class.
Finally, as we saw in a previous chapter, it would be much “cleaner and simpler” to use “default parameter” concept rather than secondary constructors!
Delegation to primary constructor
In kotlin, each secondary constructor needs to delegate to the primary constructor! This is the main rule!
If you defined a primary constructor alongside with one or more secondary constructors in your class and you want to create an object using a secondary constructor, then this secondary constructor must delegate the initialization work to the primary constructor.
You should also know that, each secondary constructor can define all primary constructor properties + its own properties.
Every secondary constructor must have a unique signature, you can’t replicate or use the same signature of the primary constructor!
Init block and secondary constructor
Like we have already mentioned before, secondary constructors are not meant for declaring class variables! To be able to use class properties (the ones that are not declared in primary constructor) inside the secondary constructor, you have to declare them inside the class body!
init blocks are always executed just after the primary constructor. So technically, initializers code is executed before the secondary constructors!
Let’s take a close look at the following example:
// class name + primary constructor + one class property : str
class Example (var str:String){
// inline class variable : strr
var strr:String=""
// init block
init {
this.str += "init"
}
// secondary constructor
constructor (str:String,strr:String) : this(str){
this.str += strr
}
}
fun main() {
val exmpl = Example("foo","boo")
println(exmpl.str)
}
If you run the above program, you will get following output : “fooinitboo”.
“foo” value is assigned to str variable using primary constructor, then the execution of init block added “init” value to the str property.
Just after the initializer block, the secondary constructor of the class is executed appending “boo” value, this is why the final value become “fooinitboo”.
Kotlin constructor and inheritance
Inheritance is at the heart of object-oriented programming! It is one of the core concepts which provide code reusability!
Inheritance describes a logical and hierarchical relation between classes! It enables a new class (subclass) to inherit the properties and methods of an existing class (superclass).
Kotlin like Java, doesn’t allow multiple inheritance of state! A kotlin class can’t extend multiple classes at the same time!
Every class in Kotlin inherits implicitly from a superclass called: Any.
All classes in Kotlin are final by design. So to make a class inheritable, you need to declare it with the open modifier. That way, you tell the compiler that this class can be derived!
You can use : to specify that a class inherits from another class! The following example shows how to define inheritance in Kotlin:
open class Animal (var color:String){
fun sound(){
print("Animal sound : ")
}
}
class Dog (color:String) : Animal(color){
fun woof(){
super.sound()
println("woof woof woof : "+this.color+" Dog")
}
}
class Cat (color:String) : Animal(color){
fun meow(){
super.sound()
println("meow meow meow : "+this.color+" Cat")
}
}
fun main() {
val d = Dog("Brown")
d.woof()
val c = Cat("Black")
c.meow()
}
// Program output:
Animal sound : woof woof woof : Brown Dog
Animal sound : meow meow meow : Black Cat
Inheritance is easy to implement but difficult to manage! You have to deal with the fact that the superclass needs to be constructed before your derived class!
Kotlin defines some simple rules to manage inheritance, the following are some key points to keep in mind when implementing inheritance!
Primary constructor and inheritance
If your base class has an explicit primary constructor, then your child class must call it!
How a derived class calls the base class primary constructor depends on whether or not it has also an explicit primary constructor.
If the drived class has its own primary constructor, then the base primary constructor must be called with its parameters: Employee subclass.
If the derived class has only secondary constructors and doesn’t define a primary constructor, then each secondary constructor has to call one of the superclass primary constructor using the super keyword or delegate to another constructor which does that! Developer subclass.
// Base class Person
open class Person (var name:String){
}
// Child class Employee with a primary constructor
class Employee (name:String, var salary:Int) : Person(name){
fun function(){
println("Hello : "+this.name+", your salary is : "+this.salary+" $$")
}
}
// Child class Developer with only one secondary constructor
class Developer : Person{
var age:Int?=20
constructor(name:String,age:Int):super(name){
this.age = age
}
fun function(){
println("Hello : "+this.name+", your are a developer and your age is : "+this.age)
}
}
fun main() {
var emp = Employee("Karin",3000)
emp.function();
var dev = Employee("Lucas",30)
dev.function();
}
// Program output:
Hello : Karin, your salary is : 3000 $$
Hello : Lucas, your are a developer and your age is : 30
Secondary constructor and inheritance
Now, let’s see what happens when a base class doesn’t have an explicit primary constructor and has only secondary constructors!
If your derived class has its own primary constructor, then you must call the super constructor from the primary constructor of your derived class! You can’t call it from a secondary constructor via super keyword! See the below image!
If you don’t define a primary constructor in your subclass, then you have to call the superclass constructor from your derived class using super keyword.
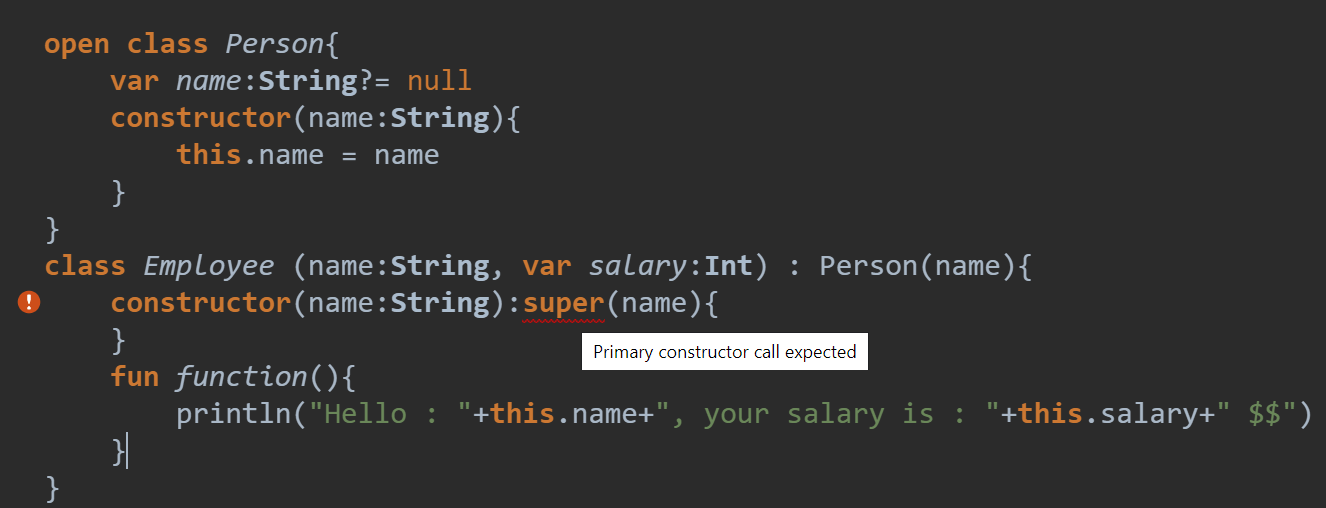
open class Person{
var name:String?= null
constructor(name:String){
this.name = name
}
}
class Employee (name:String, var salary:Int) : Person(name){
constructor(name:String):super(name){ // You CAN NOT do that !!
}
constructor(name:String):this(name,2000){
}
fun function(){
println("Hello : "+this.name+", your salary is : "+this.salary+" $$")
}
}
class Developer : Person{
var age:Int?=20
constructor(name:String,age:Int):super(name){ // You CAN do that !!
this.age = age
}
fun function(){
println("Hello : "+this.name+", your are a developer and your age is : "+this.age)
}
}
As you can see, any secondary constructor in the derived class has to call its primary constructor using this if there is one, otherwise the primary constructor of the base class using super!
If the base class doesn’t have any constructors, then the compiler creates a default one! In this case, the subclass class can call the default one from its primary constructor if it has one.
open class Person { }
class Employee (var name:String, var age:Int): Person() { }
Conclusion:
That’s all! If you are coming from a Java programming background, then you may find Kotlin syntax familiar and straightforward!
So to sum things up, you learned throughout this article, how to declare and use different types of kotlin constructor.
You learned also, that the init() block can be used to perform some initialization work just after the primary constructor is called!
I hope this tutorial will help you get started with Kotlin programming! Thank you for reading and stay tuned for the next Update!
Kotlin provide an online sandbox to explore and test your code samples directly in the browser! https://play.kotlinlang.org/