Split String Every N Characters in Java
In this short article, we are going to explore different ways to split a string every n characters in Java programming language.
First, we will start by taking a close look at solutions using Java 7 and Java 8 methods.
Then, we will shed light on how to accomplish the same thing using external libraries such as Guava.
Using Java 7 Methods
Java 7 provides multiple ways to split a particular string every n characters. Let’s take a close look at every available option.
substring Method
substring, as the name implies, extracts a portion of a string based on given indexes. The first index indicates the starting offset and the second one denotes the last offset.
Typically, we can use substring method to split our string into multiple parts every n characters.
Let’s see this method in action by writing a basic example:
public static void main(String[] args) {
String myString = "Hello devwithus.com";
int nbrOfChars = 5;
for (int i = 0; i < myString.length(); i += nbrOfChars) {
String part = myString.substring(i, Math.min(myString.length(), i + nbrOfChars));
System.out.println("Portion: " + part);
}
}
Now, let’s investigate a little bit our example. As we can see, we iterate through myString and extract every nbrOfChars characters in a separate substring.
Please note that Math.min(myString.length(), i + nbrOfChars)
returns the remaining characters at the end.
Pattern Class
Pattern class represents a compiled regular expression. In order to achieve the intended purpose, we need to use a regex that matches a specific number of characters.
For instance, let’s consider this example:
public static void main(String[] args) {
String myString = "abcdefghijklmno";
int nbr = 3;
Pattern pattern = Pattern.compile(".{1," + nbr + "}");
Matcher matcher = pattern.matcher(myString);
while (matcher.find()) {
String part = myString.substring(matcher.start(), matcher.end());
System.out.println("Portion starting from " + matcher.start() + " to " + matcher.end() + " : " + part);
}
}
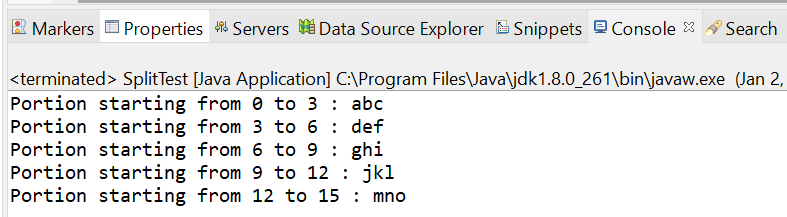
The regex .{1,n}
denotes at least one character but not more than n characters.
The idea is to create a Matcher object for the pattern and use it to match our string against our regex: .{1,n}
.
As shown above, we used substring for each matcher found to extract every portion of string starting from matcher.start() to matcher.end().
split Method
Similarly, we can use split method to answer our central question. This method relies on the specified delimiter or regular expression to divide a string into several subregions.
The best way to split a string every n characters is to use the following positive lookbehind regex: (?<=\G…)
, where … denotes three characters.
Now, let’s illustrate the use of the split method with a practical example:
public static void main(String[] args) {
String myString = "foobarfoobarfoobar";
String[] parts = myString.split("(?<=\\G...)");
for (String part : parts) {
System.out.println("Portion : " + part);
}
}
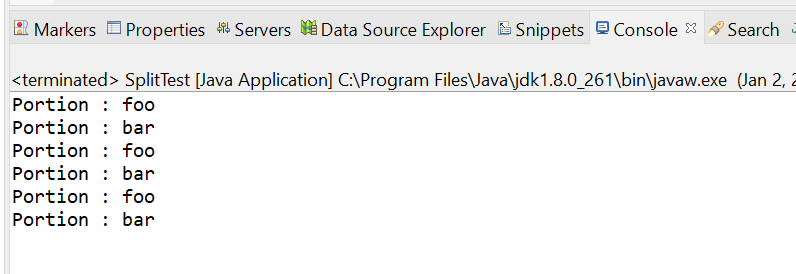
Please bear in mind that we can replace the three dots with ”.{3}”. If we want to make the number of characters dynamic, we can specify: (?<=\G.{n})
Using Java 8
Java 8 offers a another great alternative to divide a string every certain number of characters.
The first step consists on calling the chars method. Then, with the help of the Stream API, we can map and group every character using AtomicInteger class and a collector.
Please note that Collectors.joining() is a collector that concatenates chars into a single string.
public static void main(String[] args) {
AtomicInteger nbrOfChars = new AtomicInteger(0);
String myString = "zzz111aaa222ddd333";
myString.chars()
.mapToObj(myChar -> String.valueOf((char) myChar))
.collect(Collectors.groupingBy(myChar -> nbrOfChars.getAndIncrement() / 3, Collectors.joining()))
.values()
.forEach(System.out::println);
}
Output:
zzz
111
aaa
222
ddd
333
Using Guava Library
Guava is a handy library that provides a set of ready-to-use helper classes. Among these utility classes, we find the Splitter class.
Splitter provides the fixedLength() method to split a string into multiple portions of the given length.
Let’s see how we can use Guava to split a String object every n characters:
public static void main(String[] args) {
String myString = "aaaabbbbccccdddd";
Iterable parts = Splitter.fixedLength(4).split(myString);
parts.forEach(System.out::println);
}
Output:
aaaa
bbbb
cccc
dddd
Conclusion
To sum it up, we explained in detail how to split a string at every nth character using Java 7⁄8 methods.
After that, we showed how to accomplish the same objective using the Guava library.