Java Enhanced For Loop with Examples
In this article, we are going to put the focus on Java enhanced for loop.
First, we will shed light on what an enhanced for is in Java. Then, we will exemplify its use through practical examples.
Enhanced For Loop in Java
Before diving deep into the details, please bear in mind that an enhanced for loop is called also For-each in Java.
The concept of For-each is mainly introduced in Java 5 to provide a concise and convenient way to iterate over arrays and collections in a fail-safe manner.
The following is the general syntax of an enhanced for loop:
for (T item : elements_of_type_T)
{
//custom code
}
The code of For-each is compact, straightforward, and easy to understand. It makes the loop more concise and easy to read.
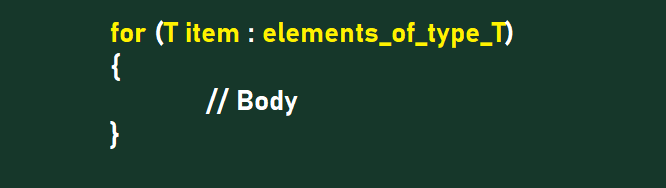
elements_of_type_T denotes a collection or an array
item represents each element of the array/collection
T describes the data type of the elements
The : in the syntax can be read as in: For each element IN the list.
The custom code specified inside the For-each loop will be executed for each element.
Iterating Over an Array using For-each
Now that we know what an an enhanced for loop is in Java, let’s see how we can use it to print the elements of a particular array:
public class EnhancedForLoop {
public static void main(String[] args) {
String[] names = { "Charlotte", "Theodore", "Abderrahim", "Violet" };
for (String name : names) {
System.out.println(name);
}
}
}
## Output:
Charlotte
Theodore
Abderrahim
Violet
As shown above, For-each offers a brand new way to print elements. We don’t need to use an explicit counter as an index to traverse our array.
In the first iteration, name will be Charlotte
In the second iteration, name will be Theodore
In the third iteration, name will be Abderrahim
In the fourth iteration, name will be Violet
Traversing Collection using Enhanced For Loop
The enhanced loop can be used also to iterate through a collection of elements. Let’s see how we can do this in practice:
Collection<Integer> ages = new ArrayList<Integer>();
ages.add(18);
ages.add(30);
ages.add(60);
int agesSum = 0;
for (Integer age : ages) {
agesSum += age;
}
System.out.println("Sum of ages is: " + agesSum);
As we can see, For-each adds each age of the collection to the agesSum variable in each iteration of the loop.
Printing Map Keys using For-each
Similarly, we can use For-each as a simple alternative to the classic for loop to manipulate Maps.
The following example illustrates how to use the enhanced for loop to print all the keys of a given Map in Java:
public static void main(String[] args) {
Map personAges = new HashMap();
personAges.put("Oliver", 18);
personAges.put("Charlotte", 41);
personAges.put("Noah", 30);
personAges.put("Emma", 25);
for (String name : personAges.keySet()) {
System.out.println("name: " + name);
}
}
As shown above, For-each is straightforward and makes our code easy to understand compared to the traditional for loop.
Difference Between Standard and Enhanced For
Now, let’s uncover the main differences between the two loops using practical examples.
To keep things simple, we will assume that we want to print an array of cities.
First, we are going to use the traditional for loop to iterate over our array:
public static void main(String[] args) {
String[] cities = { "Paris", "Manchester", "Tamassint", "Tokyo", "Abuja", "Madrid" };
for (int i = 0; i < cities.length; i++) {
System.out.println(cities[i]);
}
}
The traditional loop uses an index to traverse the array, which is not readable and difficult to understand.
Furthermore, the index can lead to ArrayIndexOutOfBoundsException if it is negative or greater than or equal to the actual size of the array.
Now, let’s acheive the same objective using the for-each loop:
public static void main(String[] args) {
String[] cities = { "London", "Brussels", "Moscow", "Canberra", "Stockholm" };
for (String city : cities) {
System.out.println(city);
}
}
As we can see, the code has reduced significantly. Besides, with the enhanced for loop, we don’t need to worry about the increment and the index.
On other hand, unlike the traditional for loop, we cannot configure for-each loop to traverse a list or an array backward.
For Each in Java 8
Java 8 comes with a great feature called forEach() which allows us to iterate over a collection in a clear and concise way.
We can use this method as an alternative to the enhanced for loop:
public static void main(String[] args) {
List<String> countries = Arrays.asList("Italy", "Poland", "Brazil", "Nigeria", "Germany");
countries.forEach(System.out::println);
}
## Outout
Italy
Poland
Brazil
Nigeria
Germany
Conclusion
To sum it up, we covered in-depth everything we should know about java enhanced for loop.
First, we explained what an enhanced for loop is. Then, we showcased how to use it in practice.
Lastly, we have discussed the main difference between a regular for and For-each loop in Java.